What is a programming paradigm?
The model or map of how we see the real world, that is a paradigm, it is a way of seeing and doing things. Following this logic, a programming paradigm is nothing more than a way of viewing and creating programming code.
Paradigms are powerful because they create the glasses or lenses through which we see the world.
Stephen R. Covey
There are three main programming paradigms used today and in JavaScript they have always existed since its first version.
- Structured programming paradigm
- Object-oriented
- Functional
Now, if we stick to the phrase of Stephen R. Covey, that paradigms create the lenses through which we see the world. Let's imagine that the three previous paradigms, each, are a pair of glasses that we put on when programming and that we can change those glasses according to our visual needs (for programming or problem solving).
Structured programming paradigm
Origin
In 1954 the FORTRAN programming language appeared, then in 1958 ALGOL and in 1959 COBOL. Time after Edsger Wybe Dijkstra in 1968 discover the structured programming paradigm, we say “discover” because they didn't actually invent it. Although this programming paradigm was formalized some time after the appearance of these programming languages, it was possible to do structured programming in them.
Dijkstra He recognized that programming was difficult, and programmers don't do it very well, which I totally agree with. A program of any complexity has many details for the human brain. In fact, the Neuroscience tells us that the focused mind It can only work with four pieces of data at a time, at most. For this reason, if one small detail is neglected, we create programs that seem to work well, but fail in ways you never imagine.
Evidence
Dijkstra's solution was to use tests, only these tests used a lot of mathematics, which was quite difficult to implement. During his research he found that certain uses of GOTO made it difficult to decompose a large program into smaller pieces, it could not be applied “divide and conquer”, necessary to create reasonable evidence.
Sequence, selection and iteration

Böhm and Jacopini They proved two years earlier that all programs can be built by just three structures: sequence, selection and iteration. Dijkstra already knew these three structures and discovered that these are the ones necessary to make any part of the program tested. This is where the structured programming paradigm is formalized.
Edsger tells us that it is bad practice to use GOTO
and suggests better using the following control structures:
- If, then, else (selection)
- do, while, until (iteration or repetition)
Decomposition of small units easy to test
Nowadays most programming languages use structured programming, JavaScript uses this type of programming. The simplest example is if conditions.
const EDAD_MINIMA = 18;
if (edad >= EDAD_MINIMA) {
// hacer algo porque es mayor que 18
} else {
// Hacer otra cosa en caso contraio
}
And if we isolate it in a function, we have a decomposition of functionalities or units that can be tested more easily. In the next section we will see the importance of having a completely probable unit
const EDAD_MINIMA = 18;
functions esMayorEdad(edad) {
if (edad >= EDAD_MINIMA) {
return true;
} else {
return false;
}
}
With this condition we have direct control of what can happen if the age is greater than or equal to 18
, and also in the event that the age is younger.
Let's not be so strict either, surely the GOTO ruling has its merits and perhaps it was very efficient in some cases, but I think that to avoid misuses that probably caused disasters, Dijkstra recommended to stop using GOTO.
Test, divide and conquer
Structured programming allows us to apply the “divide and conquer” philosophy, because we allows you to create small unit tests, until the entire program is covered. The mathematical solution of Wybe Dijkstra It was never built, precisely because of its difficulty in implementation. And unfortunately today there are still programmers who do not believe that formal tests are useful for create high quality software.
And I say create, because verify correct operation after create, is nothing more than a simple measurement and the opportunity to reduce time and money is lost.
Scientific method, experiments and impiricism
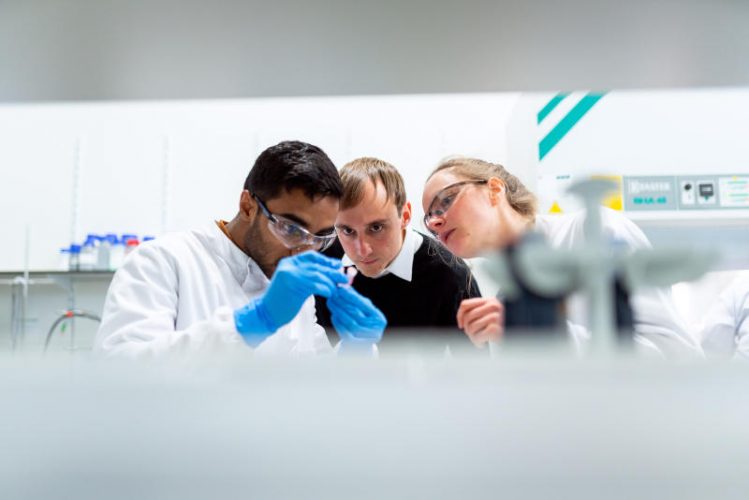
The good news is that the mathematical method is not the only way to verify our code, we also have the scientific method. Which cannot prove that things are absolutely correct from a mathematical point of view. What we can do is create experiments and obtain enough results to verify that our theories work. Since our theories work on these experiments, then we conclude that they are “correct” enough for our validation purposes.
If the theory is easily validated as false, then it is time to modify or change it. This is the scientific method and this is how tests are currently done:
First we establish our theory (write the test), then we build the experiments (write production code) and finally we verify (run the test) and repeat this cycle until we have enough evidence.
Doesn't this sound like a current agile practice called TDD?
Tests show the presence, not the absence, of defects
Edsger Wybe Dijkstra said “Testing shows the presence, not the absence, of defects.” That is, a program can be proven wrong by testing, but it cannot be proven correct. All we can do with our tests is validate that our program works well enough for our goals. But we can never be one hundred percent sure of the absence of defects.
If we can never be one hundred percent sure that our code is correct, even with its tests, what makes us think that without tests we deliver a program with sufficient validity to affirm that no negligence is consciously committed?
direct control
lto structured programming teaches us about direct control, that is, controlling the sequential flow of operations through the control structures, without neglecting the recommendation of Wybe Dijkstra about GOTO. This recommendation also applies to statements that drastically change the normal flow of operations, for example a break
within a cycle for
nested, that break
It breaks direct control because it abruptly complicates the understanding of the algorithm. Let's not be purists either, there will surely be cases where you need these sentences, but in the first instance, try to avoid them.
In conclusion, the structured programming paradigm teaches us:
The direct, clear and explicit control of what a piece of code does
Object-oriented programming paradigm
Arised from functions?
The object-oriented programming paradigm was discovered in 1966 by Ole Johan Dahl and Kristen Nygaard when they were developing simula 67. Two years before structured programming and its adoption by the programming community was some time later. In 1967 it was launched Simulates 67, Simulates It was the first object-oriented programming language, it basically added classes and objects to ALGOL, initially Simula was going to be a kind of extension of ALGOL.
During the development of Simula, it was observed that the execution of an ALGOL function requires certain data, which could be moved to a tree structure. This tree structure is a Heap.
The heap allowed you to declare local variables of the function that can exist even after the function returns a value. Doesn't this last sound like what closure is in JavaScript?
The parent function becomes a constructor, the local variables become the properties, and the child (nested) functions become its methods. In fact, this pattern is still widely used by Javascript to create modules and use functional inheritance. This also describes how classes work in Javascript, behind are functions.
Spread of polymorphism
All this helped a lot to implement polymorphism in object-oriented programming languages. Ole Johan Dahl and Kristen Nygaard invented the notation:
object.function(parametro)
The execution of function
that belongs to object
, but more importantly, we pass a message through parameters from the first part of the code to the second part located in a different object.
Molecular biology and message passing
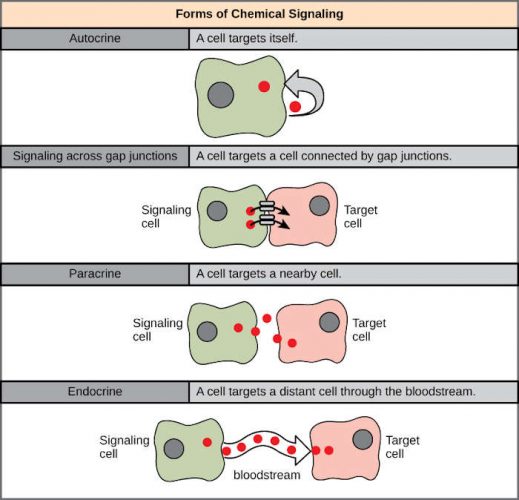
Some time later it was created Smalltalk, a much more sophisticated and modern object-oriented programming language, in charge of this project was Alan Kay. This person is credited with the formal definition of object-oriented programming.
The main influence on Alan Kay's object-oriented programming was biology, he has a degree in biology. Although it is obvious that it was influenced by Simula, the influence of LISP (functional programming language) is not so obvious.
From the beginning he thought of objects as interconnected cells in a network, from which they could communicate through messages.
Alan Kay commented:
I'm sorry I coined the term a long time ago. Objects for programming because it made people focus on the least important part. The big idea is “Sending messages“.
Alan Kay
My English is bad so you can check the original text here.
Better object composition over class inheritance
Smalltalk It also allowed the creation of Self, created at Xerox Parc and later migrated to Sun Microsystems Labs. It is said that Self It is an evolution of smalltalk.
In this programming language the idea of prototypes, eliminating the use of classes to create objects, this language uses the objects themselves to allow one object to reuse the functionalities of another.
Self It is a fast language and is generally known for its great performance, self It did a good job on garbage collection systems and also used a virtual machine to manage its execution and memory.
The virtual machine Java HotSpot It could be created thanks to Self. Lars Bak one of Self's latest contributors, he was in charge of creating the V8 Javascript engine. Due to the influence of Self we have today the engine of JavaScript V8, which uses it node.js, mongoDB and Google Chrome internally.
Self followed one of the book's recommendations Design Patterns: Elements of Reusable Object-Oriented Software, long before it came out, published this recommendation:
Better composition of objects to class inheritance.
Design Patterns: Elements of Reusable Object-Oriented Software
Example
Currently Javascript uses prototypes for code reuse, you could say that it uses composition, instead of inheritance. Let's look at an example:
const person = {
greet () {
return 'Hola'
}
};
// se crea un programador con el prototipo igual al objeto persona
const programmer = object.create(persona);
programmer.programar = () => 'if true';
const saludo = programmer.greet();
const codigo = programmer.programar();
console.log(saludo); // 'Hola'
console.log(codigo); // 'if true'
The object programmer
It is based on the prototype of `person`. It doesn't inherit, it just directly reuses the functionality of `person`.
JavaScript emerged shortly after JAVA, in the same year. JavaScript took influences from Self, and in turn Self was influenced by smalltalk.
The syntax of current classes in Javascript is nothing more than a facade, well a little more accurately they are an evolution of the constructor functions, but internally, their basis are functions and prototypes.
Communication with message passing
As we already saw, the origin of object-oriented programming has its origins in functions, and the main idea has always been communication between objects through message passing. We can say that Object-oriented programming tells us of message passing as a key piece for the communication of objects, for example, when a method of one object invokes the method of another.
object.function(parametro);
In conclusion, the object-oriented programming paradigm teaches us:
Message passing for communication between objects
Functional programming paradigm
lambda calculation
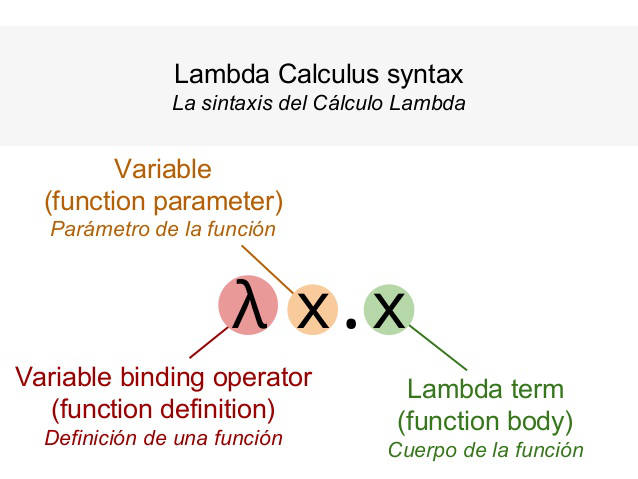
Functional programming is the oldest programming paradigm, in fact its discovery was long before computer programming, it was discovered in 1936 by Alonzo Church, when I invented the lambda calculus, based on the same problem to be solved by your student Alan Turing.
As a curious fact, the symbol of the lambda calculus is:
λ
The first language based on functional programming was LISP, created by John McCarthy. As we saw previously, Alan Kay also took influence from LISP to create Smalltalk, with this we can see that the programming paradigms can be united and their relationship between them arises from the idea of how to solve problems more efficiently, they are not fighting, nor Separated, they seek the same goal and somehow evolve together.
The basis of the lambda calculus is immutability, We will review this concept later.
Similarities with OO and structured paradigms
As we saw in the structured programming paradigm, we can decompose our programs into smaller units called procedures or functions. Since functional programming is the oldest paradigm, we can say that the structured programming paradigm also relies on functional programming.
Now, if we analyze the notation a little object.function(x)
that we saw in the object-oriented programming paradigm section, is not very different from function(object, parameters)
, these two lines below are the same, the fundamental idea is the passing of messages, as Alan Kay tells us.
object.function(parametro);
function(objeto, parametro);
Smalltalk uses the “The Actor Model”, which says that actors communicate with each other through messages.
On the other hand we have LISP, which has a “Function dispatcher model” What we currently call functional language, these models are identical, because what functions and methods do is send messages between actors.
An example of this is when a function calls another function or when an object's method is invoked, what happens is that actors exist and they communicate with each other through messages.
Message passing again
So we can emphasize that the main idea of OOP is the sending of messages and that it is not very different from functional programming, in fact according to what we already established in the previous sections, OOP was born from a functional base. And here it is important to highlight what he said Alan Kay Co-creator of SmallTalk:
I'm sorry I coined the term a long time ago. Objects for programming because it made people focus on the least important part. The big idea is “Sending messages“.
Alan Kay
From the communication models between Smalltalk and LISP messages, it was created scheme.
scheme has the goodness to use tail recursion and closure to obtain a functional language, closure allows access to the variables of an external function even when it has already returned a value. This is the same principle that gave rise to object-oriented programming by Ole Johan Dahl and Kristen Nygaard. Do you remember the closure question in Javascript?
Javascript uses closure a lot in its functional programming paradigm. JavaScript took influences from scheme, at the same time scheme was influenced by LISP.
No side effects
The basis of the lambda calculus is immutability, therefore, lImmutability is also the basis of the functional programming paradigm.
We as functional programmers must follow this principle and limit object mutations as much as possible to avoid side effects.
I repeat, the main idea of functional programming is immutability, which allows you to create your programs with fewer errors by not producing side effects that are difficult to control.
As an example, suppose we have an object person
and we want to “change” the name, the most obvious thing would be to modify the name property directly, but what happens if that object is used elsewhere and the name is expected to be “Jaime”, that is why instead of changing the name property , we only create a new person object with a different name, without modifying the original.
functions cambiarNombre(name, person) {
return {
name: name,
edad: person.edad
}
}
const James = { name: 'Jaime', edad: 30 };
const juan = cambiarNombre('Juan', jaime);
console.log(jaime); // { nombre: 'Jaime', edad: 30 }
console.log(juan); // { nombre: 'Juan', edad: 30 }
Here you will find more details about the fundamental principles of functional programming:
Introduction to functional programming
Finally, the functional programming paradigm teaches us:
No side effects, for clear expression and less prone to errors
Conclusion
It is important to note that if the three programming paradigms were implemented between 1958 with LISP (functional programming) and Simula (object-oriented programming) in 1966, and the discovery of the structured programming paradigm in 1968, in only a period of 10 For years there has been innovation in programming paradigms, and new paradigms have not really emerged, unless they are based on these three main ones.
Right now more than 60 years have passed, which tells us the importance and firmness they have despite the time that has passed.
- Functional programming paradigm (1958, already implemented in a programming language for computers, remember that it was discovered in 1936)
- Object Oriented (1966)
- Structured (1968)
The implementation of these three paradigms from the beginning within JavaScript has made this programming language the global success it is today. And it proves to us the value of combining paradigms when writing our programs.
JavaScript is a multi-paradigm programming language, so you can combine the paradigms to create much more efficient and expressive code using:
- The direct, clear and explicit control of what a piece of code does
- Message passing for communication between objects
- No side effects, for clear expression and less prone to errors
References
https://en.wikipedia.org/wiki/Structured_programming
https://en.wikipedia.org/wiki/Structured_program_theorem