Variables
One of the most used elements are variables in Javascript. We can describe variables in Javascript as boxes to hold information, which we label so that we can find that information easily. This label is the name of the variable and the box is the space used in RAM.
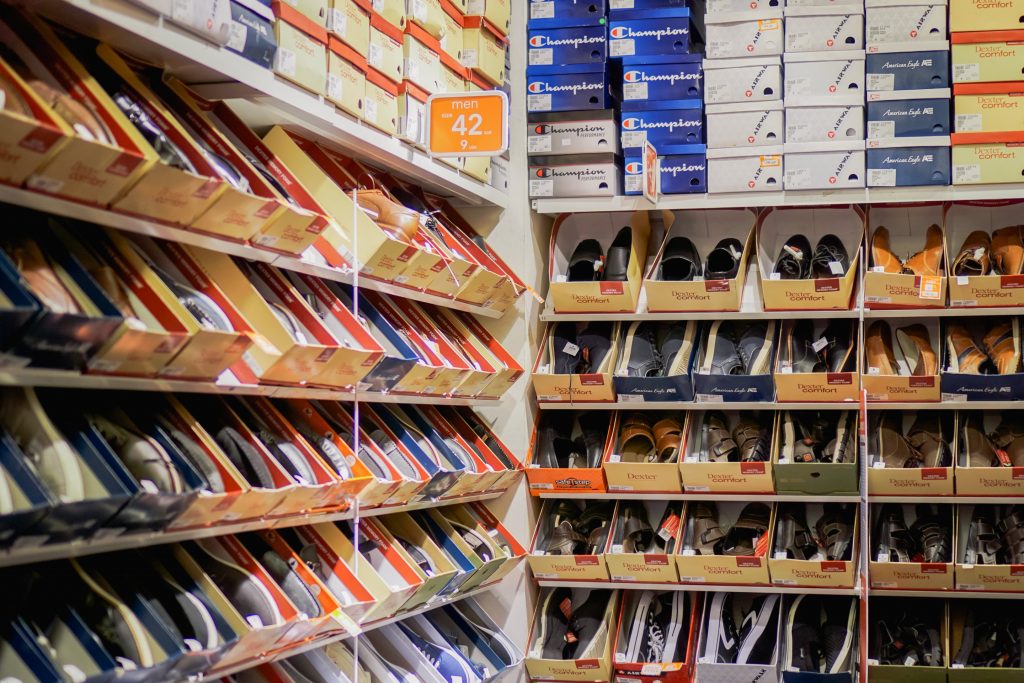
This is what variables are like in Javascript, and in fact in any programming language. You can also store any datatype, whether they are numbers, text strings, functions or any type of object.
There are two ways to declare a variable. One is using let and the other using var.
//Variables de tipo String
var name = 'Jaime';
let last name = "Cervantes"
The shape var
It is the one that has always existed since the origin of language, then in 2015 it appeared let
in order to mitigate certain deficiencies of var
. For this reason, the recommendation is to use let
In any case, it is important to know well var
because you will come across a lot of code that still uses var
.
How are variables used in Javascript?
The name or identifier of a variable, it cannot start with a number, it must start with some letter (including _ and $), and Javascript is also case-sensitive. Is not the same name
and Name
, these are two different variables.
Variables are defined in two ways:
With a single let
:
let name = 'Jaime',
last name = 'Cervantes',
mother's last name = 'Velasco';
a single var
:
var name = 'Jaime',
last name = 'Cervantes',
mother's last name = 'Velasco';
Now with a let for each variable:
let name = 'Jaime';
let last name = 'Cervantes';
let mother's last name = 'Velasco';
With a var
for each variable.
var name = 'Jaime';
var last name = 'Cervantes';
var mother's last name = 'Velasco';
In the previous example we define variables of type string
(text strings), but remember that you can store any type of data.
It is advisable to put each variable on its own line because it is faster to understand for the “you” of the future and your colleagues who have not touched your code. For example, DO NOT define the variables like this:
let name = 'Jaime', last name = 'Cervantes', mother's last name = 'Velasco'; edad = 32, sexo = 'H'
Let's try numbers.
let edad = 32.9;
let hijos = 1;
var edad = 32.9
var hijos = 1;
Boolean data only stores false or true.
let esHombre = true;
let esAlto = false;
var esHombre = true;
var esAlto = false;
As its name says, its value can be variable, therefore you can reassign a new value to your variable:
var name = 'Jaime';
name = 'Pepito';
name = 'Menganito';
console.log(nombre); // 'Menganito'
Variable scopes in Javascript, let vs var
The main difference between variables defined with let
and var
is the scope they reach when they are declared:
- With
let
you have block scope{}
. - With
var
you have scope of functionfunction()
but not block.
Example of block scope.
if (true) {
let name = 'James';
}
console.log(nombre); // Uncaught ReferenceError: nombre is not defined
Because let
has block scope, when wanting to access the variable name
Javascript shows:
Uncaught ReferenceError: name is not defined
Try to use block scope with var
.
if (true) {
var name = 'Jaime';
}
console.log(nombre); // 'Jaime';
In this example the variable name
If it can be accessed from outside the blocks, this is because it is not inside any function.
Now we are going to declare the variable with var
inside a function.
functions setearNombre() {
var name = 'Jaime';
}
setearNombre();
console.log(nombre); // Uncaught ReferenceError: nombre is not defined
Let's see what happens with let
when we try to use function scope.
functions setearNombre() {
let name = 'Jaime';
}
setearNombre();
console.log(nombre); // Uncaught ReferenceError: nombre is not defined
If we define a variable with let
inside a function, we can't access it from outside either, because the function definition itself uses blocks {}
.
Constants in Javascript
Constants are in the same way as variables, labeled boxes to store information, with the only difference that they cannot be reassigned to any other value.
const name = "Jaime";
name = "Pepito"; // Uncaught TypeError: Assignment to constant variable
Let's not forget to declare each constant on its own line to improve the readability of our code.
const name = "Jaime";
const last name = "Cervantes";
const mother's last name = 'Velasco';
const name = 'Jaime',
last name = 'Cervantes',
mother's last name = 'Velasco';
Same rules as variables with let
Constants follow the same block scope rules as variables in Javascript with let
.
As well as let
, const
has block scope, so if you define a variable with var
and has the same name as a constant, the following error will be thrown:
{
const name = "Jaime";
var name = "Pepito"; // Uncaught SyntaxError: Identifier 'nombre' has already been declared
}
Constants in javascript read-only and mutable
Constants are read-only, which is why they cannot be reassigned a value. Even so, these saved values continue to preserve the behavior of their data type.
For example, if you create a constant for an object, you can change the properties of that object.
An object literal in JavaScript is nothing more than a set of values identified with a name or key, like the following:
const James = {
name: "Jaime",
last name: "Cervantes",
mother's last name: "Velasco"
};
James.name = "Pepito"; // todo bien, se puede cambiar una propiedad
James = {}; // Uncaught Type Error: Assigment to constant variable
The reference to the object is read-only, but it does not mean that the value is immutable. As seen in the example, you can change the property, but not reassign a new value to the constant James
as we can see in the last line of the example.
How to name variables and constants in Javascript?
Any fool can write code that a computer can understand. Good programmers write code that humans can understand.
Martin Fowler
The main objective of naming variables and constants is to immediately understand what we are storing in them. It is important to correctly describe the content of the variables, because this makes life easier for the next person who needs to read and modify our code.
This next person is very often “you” from the future, and unless you are a robot, you won't be able to remember every detail of your code.
Most of the time spent by a programmer is reading and understanding code, we don't want to spend a lot of time deciphering the content of them, as programmers we are happier creating new things.
For this reason we should not be surprised by the importance in naming our variables and constants, it is one of the best ways to not waste our time and the time of our colleagues.
Recommendations:
- Take enough time to name a variable. It's like organizing your room, the tidier it is, the faster it will be to find the other sock (it happens to me a lot when I don't organize mine)
xd
. - The name must be very semantic and explain its context. Avoid names like
data
eitherinfo
because they don't say much about the context, it is obvious that they are data and information, but for what? or what?. - Agree with your colleagues on how to name variables, especially those that are closely related to the business. For example, for a user's registration data, it can be “UserRegistration”, “CustomerRegistration”, “VisitorRegistration”, all three forms can be valid (it depends a lot on the context), but it is better to follow a convention through an agreement.
- Avoid very short names like “u1”, “a2”, “_”, “_c”
- Avoid abbreviations, prefixes, suffixes and infixes. These are difficult to understand. For example
pmContent
What is “pm”? - If the variable or constant has a very small scope, that is, it is used in nearby lines, then the name can be short.
- If the variable or constant is used in a large scope, that is, it is referenced over a distance of many lines, then the name must be very descriptive, and therefore it is normal that it can be long.